5 Practical CSS variables tricks and tips
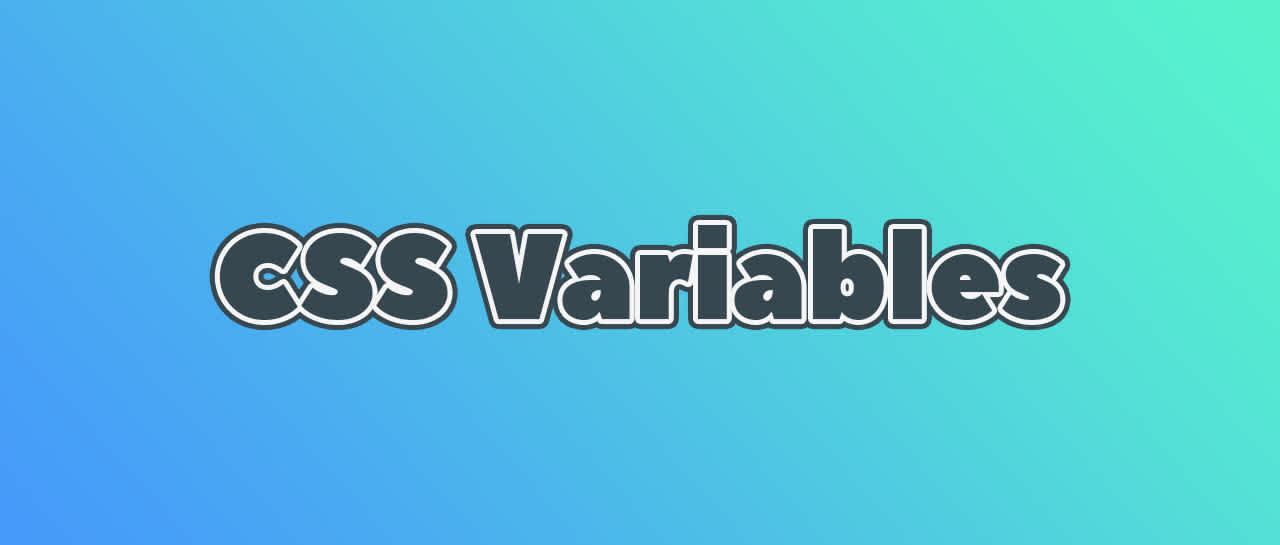
CSS doesn't have the power of Sass, such as mixins, functions, etc, but it has another secret weapon called CSS variables which can be very useful.
In this article, I don't want to compare CSS and Sass variables as you can find many posts on the internet that check their differences. However, here I would like to share some practical points and tricks that I've learned while using these custom properties. So fasten your seat belt and keep reading!😉
1. Implementing dark mode using CSS variables
To do so first, you need to add a data-theme attribute to the HTML element, like below:
<!DOCTYPE html>
<html data-theme="light">
<head>
<title>Panbehkar</title>
</head>
<body>
<h1>Sample Text</h1>
<button onclick="toggleTheme()">Toggle Theme</button>
<script>
const toggleTheme = () => {
const html = document.documentElement;
const currentTheme = html.getAttribute("data-theme");
html.setAttribute("data-theme", currentTheme === "light" ? "dark" : "light");
};
</script>
</body>
</html>
Here you should take into account that it's not mandatory to use the term "data-theme", It's just a convention. Afterward, you need to use a JavaScript function to toggle the data-theme attribute between "light" and "dark". Then you can define CSS variables like below:
:root,
[data-theme="light"] {
--background-color: #f8f8fe;
--text-color: #0c0a1f;
}
[data-theme="dark"] {
--background-color: #0c0a1f;
--text-color: #f8f8fe;
}
body {
background-color: var(--background-color);
color: var(--text-color);
}
That's it! Now you can check the result that should look like below:
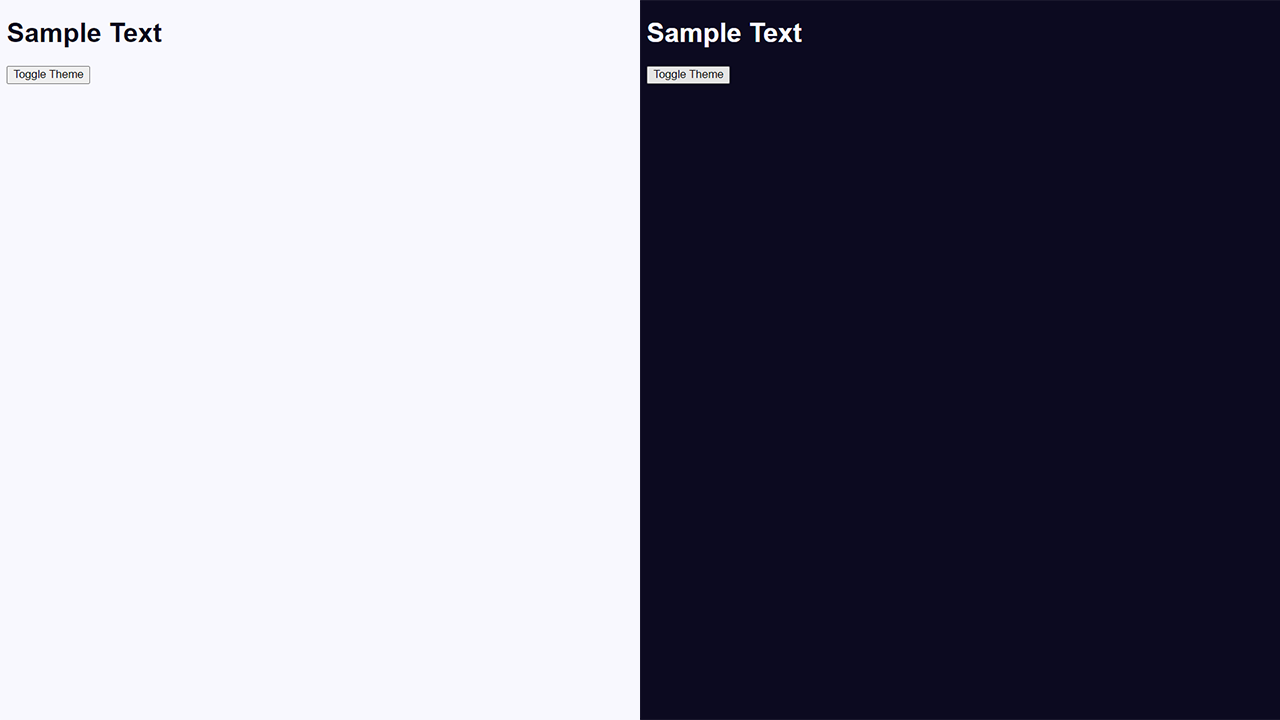
2. Using mathematical operators in CSS variables
For using mathematical operators, you can use the calc() CSS function! I'm completely aware that by using Sass it will be cleaner and easier to work with, but you know, sometimes you might be interested and limited to just using native CSS without being dependent on any extra packages or going through the complexity of installing additional dependency.
In the following example, you can see how to use the logical operators just by using CSS variables:
:root {
--margin: 20px;
}
.item {
margin-top: calc(var(--margin) / 2); /********* 10px */
margin-right: calc(var(--margin) * 0.5); /***** 10px */
margin-bottom: calc(var(--margin) - 5px); /**** 15px */
margin-left: calc(var(--margin) * -1); /****** -20px */
}
3. Generating shades of color using CSS variables
To achieve this, you can use these two color systems: hsl(hue, saturation, lightness) and rgba(red, green, blue, alpha).
Let's look at the following examples:
:root {
/* hsl example - base color: hsl(213, 96%, 63%) */
--primary-hs: 213, 96%; /* hue, saturation */
--primary-l: 63%; /******* lightness */
/* rgba example - base color: rgba(69, 150, 251, 1) */
--secondary-rgb: 69, 150, 251; /* red, green, blue */
--secondary-a: 1; /************** alpha channel (transparency) */
}
/* hsl */
.item-1 { /* hsl(213, 96%, 63%) */
background-color: hsl(var(--primary-hs), var(--primary-l));
}
.item-2 { /* hsl(213, 96%, 73%) */
background-color: hsl(var(--primary-hs), calc(var(--primary-l) + 10%));
}
.item-3 { /* hsl(213, 96%, 83%) */
background-color: hsl(var(--primary-hs), calc(var(--primary-l) + 20%));
}
.item-4 { /* hsl(213, 96%, 93%) */
background-color: hsl(var(--primary-hs), calc(var(--primary-l) + 30%));
}
/* rgba */
.item-5 { /* rgba(69, 150, 251, 1) */
background-color: rgba(var(--secondary-rgb), var(--secondary-a));
}
.item-6 { /* rgba(69, 150, 251, 0.8) */
background-color: rgba(var(--secondary-rgb), calc(var(--secondary-a) - 0.2));
}
.item-7 { /* rgba(69, 150, 251, 0.6) */
background-color: rgba(var(--secondary-rgb), calc(var(--secondary-a) - 0.4));
}
.item-8 { /* rgba(69, 150, 251, 0.4) */
background-color: rgba(var(--secondary-rgb), calc(var(--secondary-a) - 0.6));
}
Tadaaa! Here is the output: Isn't it beautiful ?!🤩
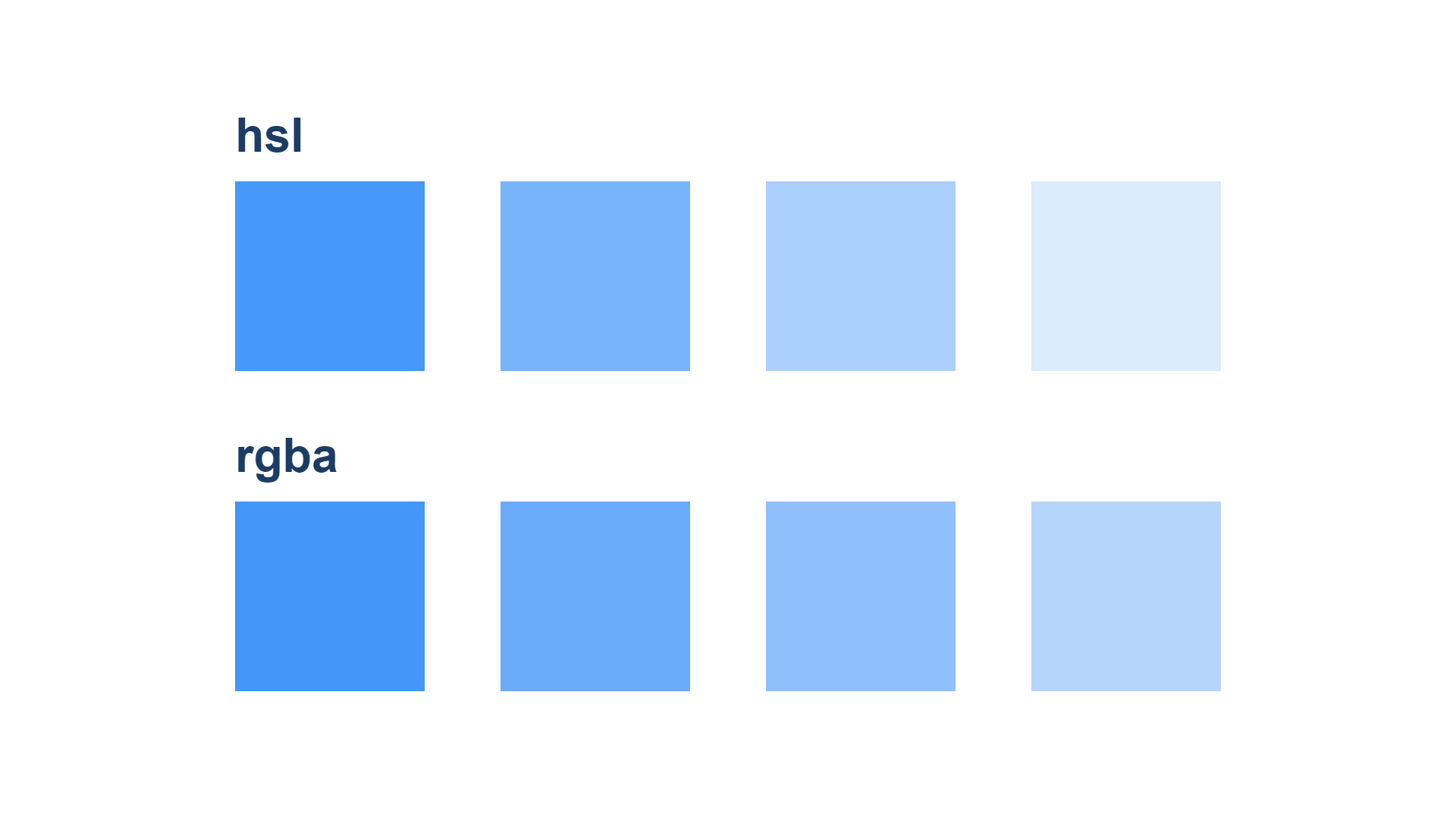
4. Update CSS variables with JavaScript
Consider the following CSS code snippet:
:root {
--box-size: 400px;
}
.box {
width: var(--box-size);
height: var(--box-size);
background-color: #57f2cc;
}
We can update CSS variables easily with the following JavaScript function:
<!DOCTYPE html>
<html>
<head>
<title>Panbehkar</title>
</head>
<body>
<button onclick="updateVariable()">Change Box Size</button>
<div class="box"></div>
<script>
const updateVariable = () => {
const html = document.documentElement;
console.log(getComputedStyle(html).getPropertyValue("--box-size")); // 400px
html.style.setProperty("--box-size", "200px");
console.log(getComputedStyle(html).getPropertyValue("--box-size")); // 200px
};
</script>
</body>
</html>
And here is the result:
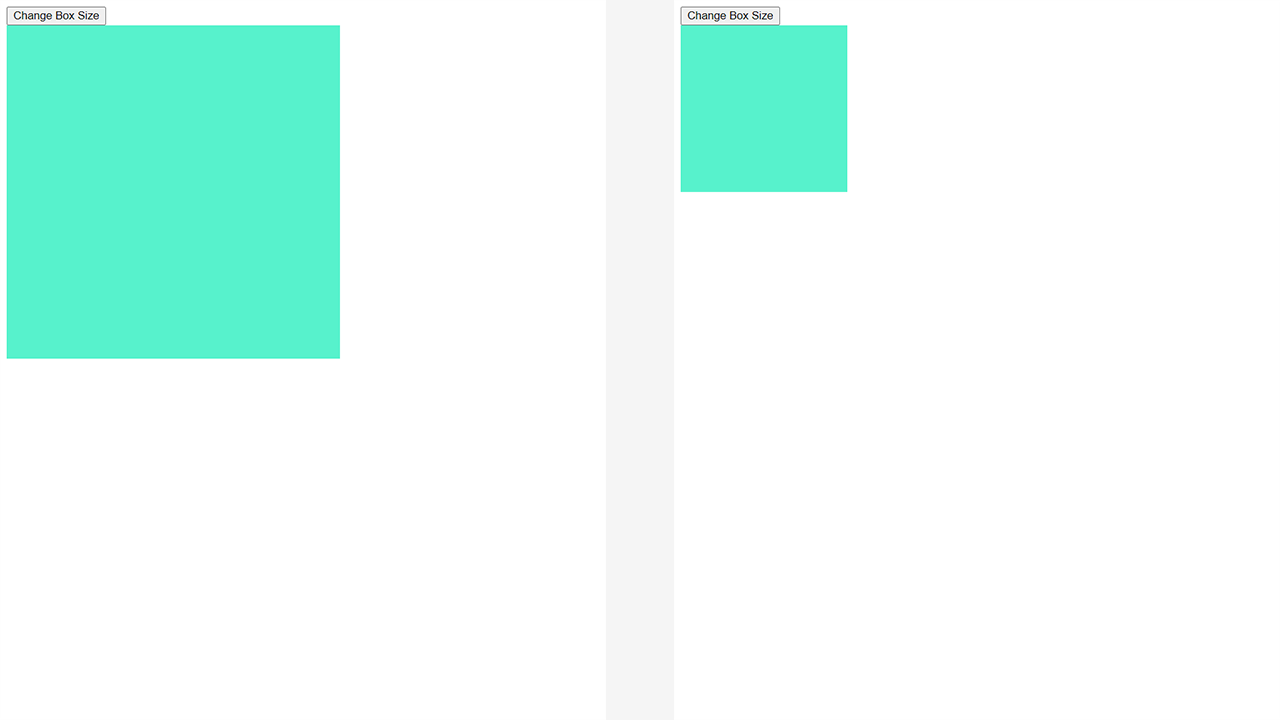
5. Categorizing CSS variables!
As the last tip, I would like to share with you a pretty funny trick that allows you to represent the CSS variables section in a more readable style. Just take into account that this is not a new feature or best practice in CSS variables. It's only an interesting idea which I come up with.
Using variables in the browser's inspector can be confusing as the number of CSS variables gets increased. So to make it easier to read, here is my little trick, by using a variable name:
:root {
--_______________Palette_______________--: ;
--primary: CornflowerBlue;
--secondary: LightSkyBlue;
--success: Green;
--danger: Red;
--warning: Orange;
--info: MediumPurple;
--dark: MidnightBlue;
--light: AliceBlue;
--_______________Typography_______________--: ;
--font-family: Arial;
--font-size-sm: 15px;
--font-size-md: 17px;
--font-size-lg: 20px;
--font-weight-thin: 300;
--font-weight-regular: 500;
--font-weight-bold: 700;
--line-height: 1.5;
--_______________MediaQueries_______________--: ;
--xs: 0;
--sm: 576px;
--md: 768px;
--lg: 992px;
--xl: 1200px;
}
Now let's see the result:
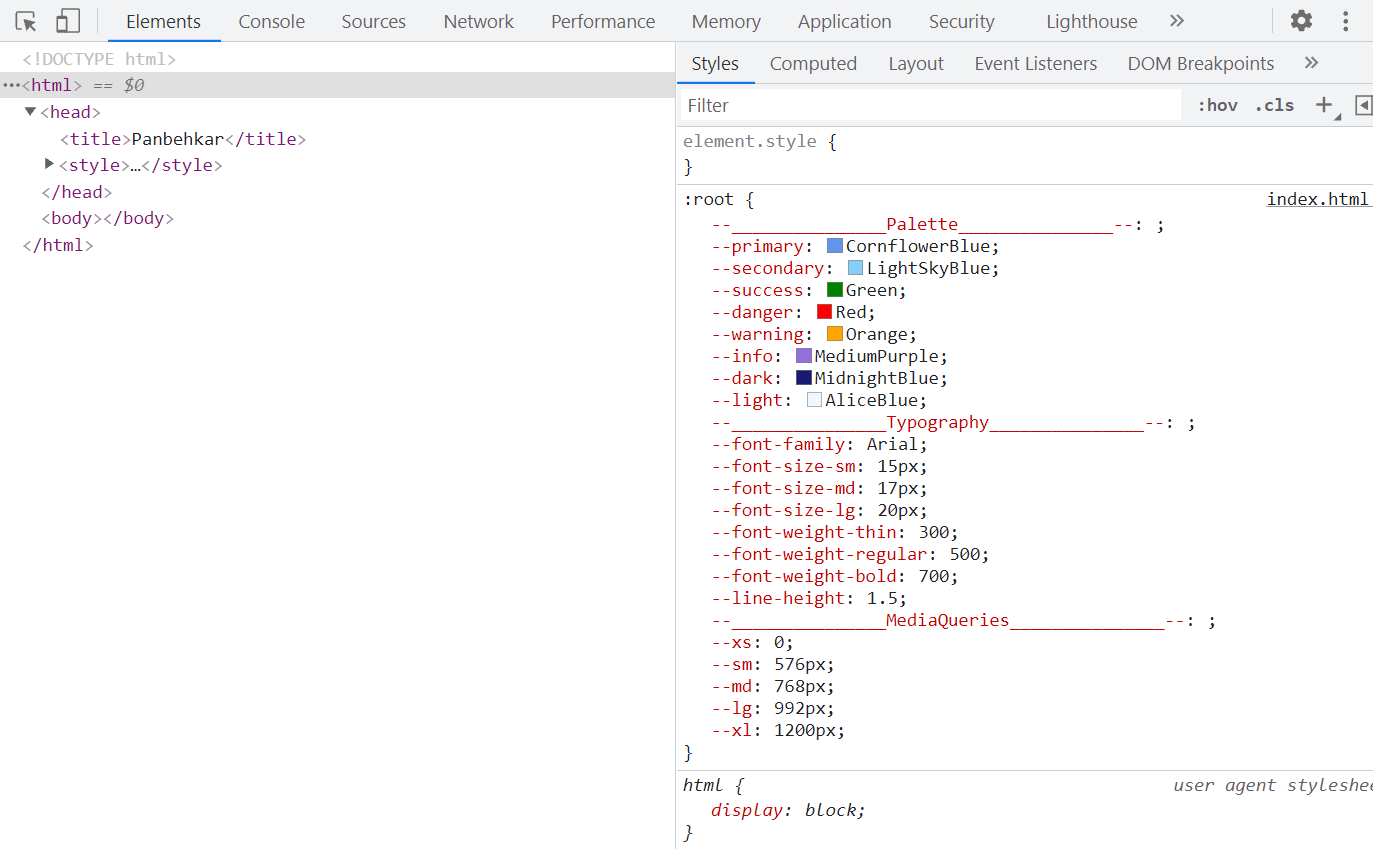
I hope you found this article helpful😊
Thanks for your attention🌺
2 years ago